Build Your Own AI Chatbot with React and OpenAI API
This tutorial guides you through building a smart, conversational chatbot using React and the OpenAI API, perfect for enhancing your web applications with AI.
TUTORIALS
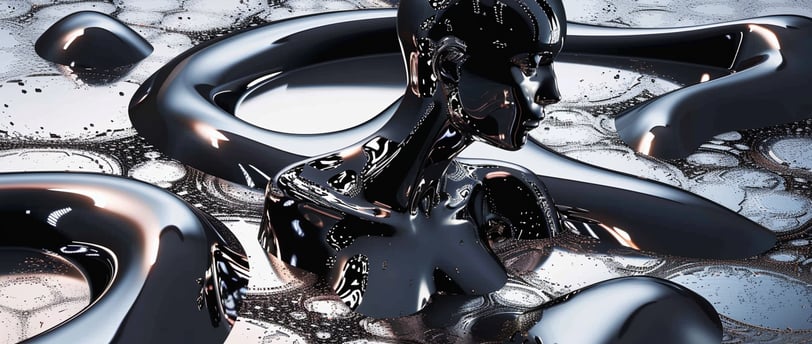
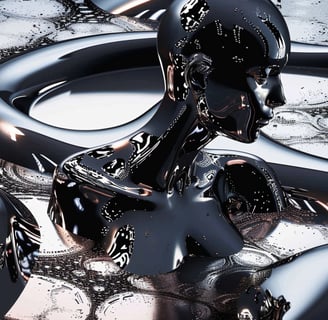
Introduction to Chatbots
Chatbots have emerged as a vital tool within the digital landscape, serving a broad array of functions across industries. At their core, chatbots are software applications that simulate human-like conversations through textual or auditory methods. Utilizing artificial intelligence (AI), these systems can understand, interpret, and respond to user queries, making them invaluable for personal and business interactions alike.
One of the primary applications of chatbots is in customer service, where they can handle a multitude of inquiries simultaneously, providing instantaneous responses to users. This capability not only enhances the customer experience but also reduces operational costs for businesses. By automating routine tasks such as answering frequently asked questions or booking appointments, companies streamline their processes and allow human agents to focus on more complex issues.
The advancements in natural language processing (NLP) have significantly increased the efficacy of AI-powered chatbots. NLP enables these systems to comprehend the nuances of human language, allowing for more natural and coherent interactions. As a result, users often experience less friction when engaging with chatbots, fostering a more engaging communication dynamic. Furthermore, the growing significance of such technology is evident in the increasing number of businesses adopting chatbots as part of their digital strategy.
In addition, chatbots can be tailored to serve various sectors, including e-commerce, healthcare, education, and entertainment, contributing to their widespread appeal. They provide valuable insights through data analytics, allowing organizations to track user behavior and preferences. This information can drive better decision-making, ultimately enhancing business strategies.
Given the rapid technological advancements and the versatility of chatbot applications, building a chatbot with modern tools like React and the OpenAI API is a highly relevant skill. As the landscape continues to evolve, understanding the fundamentals of chatbots prepares individuals for many opportunities in the tech-driven world.
Tech Stack Overview
React: For building the user interface.
OpenAI API: To provide conversational capabilities to the chatbot.
Express (optional): For securely handling API requests on the server side.
Tailwind CSS: For styling the chatbot and giving it a modern, responsive design.
Setting Up the React Project
Learn to create a smart, conversational chatbot from scratch using React, OpenAI, and modern web technologies. Empower your web applications with interactive AI capabilities!
First, let's set up a new React project. You can use create-react-app or Vite for faster development:
npx create-react-app ai-chatbot
Or, if you prefer Vite for a snappy experience:
npm create vite@latest ai-chatbot --template react
After creating the project, navigate to the directory and install dependencies:
cd ai-chatbot
npm install axios dotenv
We'll use axios for making API requests and dotenv for handling environment variables.
We'll use axios for making API requests and dotenv for handling environment variables.
Connecting to OpenAI
To interact with the OpenAI API, you'll need an API key. You can sign up and obtain your API key here: OpenAI API.
Create a .env file in your project root and add your API key:
REACT_APP_OPENAI_API_KEY=your_api_key_here
To ensure the API key isn't exposed on the client-side, we'll use a serverless function or an Express backend to handle the API calls.
For an Express setup, create a new file server.js:
const express = require('express');
const axios = require('axios');
require('dotenv').config();
const app = express();
app.use(express.json());
app.post('/api/chat', async (req, res) => {
try {
const response = await axios.post('https://api.openai.com/v1/completions', {
model: 'text-davinci-003',
prompt: req.body.prompt,
max_tokens: 100,
}, {
headers: {
'Authorization': `Bearer ${process.env.OPENAI_API_KEY}`
}
});
res.json(response.data);
} catch (error) {
res.status(500).send('Error communicating with OpenAI');
}
});
app.listen(5000, () => console.log('Server running on port 5000'));
Building the Chat UI
In your React app, create a new component called Chatbot.js. This component will handle user inputs and display chat responses.
import React, { useState } from 'react';
import axios from 'axios';
function Chatbot() {
const [messages, setMessages] = useState([]);
const [input, setInput] = useState('');
const handleSend = async () => {
const userMessage = { sender: 'user', text: input };
setMessages([...messages, userMessage]);
setInput('');
try {
const response = await axios.post('/api/chat', { prompt: input });
const botMessage = { sender: 'bot', text: response.data.choices[0].text };
setMessages((prevMessages) => [...prevMessages, botMessage]);
} catch (error) {
console.error('Error:', error);
}
};
return (
<div className="chat-container">
<div className="messages">
{messages.map((msg, index) => (
<div key={index} className={msg.sender === 'user' ? 'user-message' : 'bot-message'}>
{msg.text}
</div>
))}
</div>
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Ask me anything..."
/>
<button onClick={handleSend}>Send</button>
</div>
);
}
export default Chatbot;
Adding Personality to the Chatbot
To give your chatbot some character, you can customize the prompt sent to OpenAI. For example:
const response = await axios.post('/api/chat', { prompt: `You are a helpful and witty assistant. ${input}` });
This makes the bot more engaging and adds a unique touch to its responses.
<div className="chat-container bg-gray-100 p-4 rounded shadow-md">
{/* Your chat UI code here */}
</div>
Conclusion
You've successfully built an AI-powered chatbot using React and OpenAI's API! Not only does this project introduce you to integrating AI with web development, but it also showcases how you can enhance user engagement with conversational AI. Feel free to extend theDeploying the App
You can deploy both the frontend and backend of your chatbot with services like Vercel or Netlify. For example, Vercel makes deploying serverless functions seamless, which can be ideal for handling the API requests securely.
Happy coding and keep exploring AI's potential!